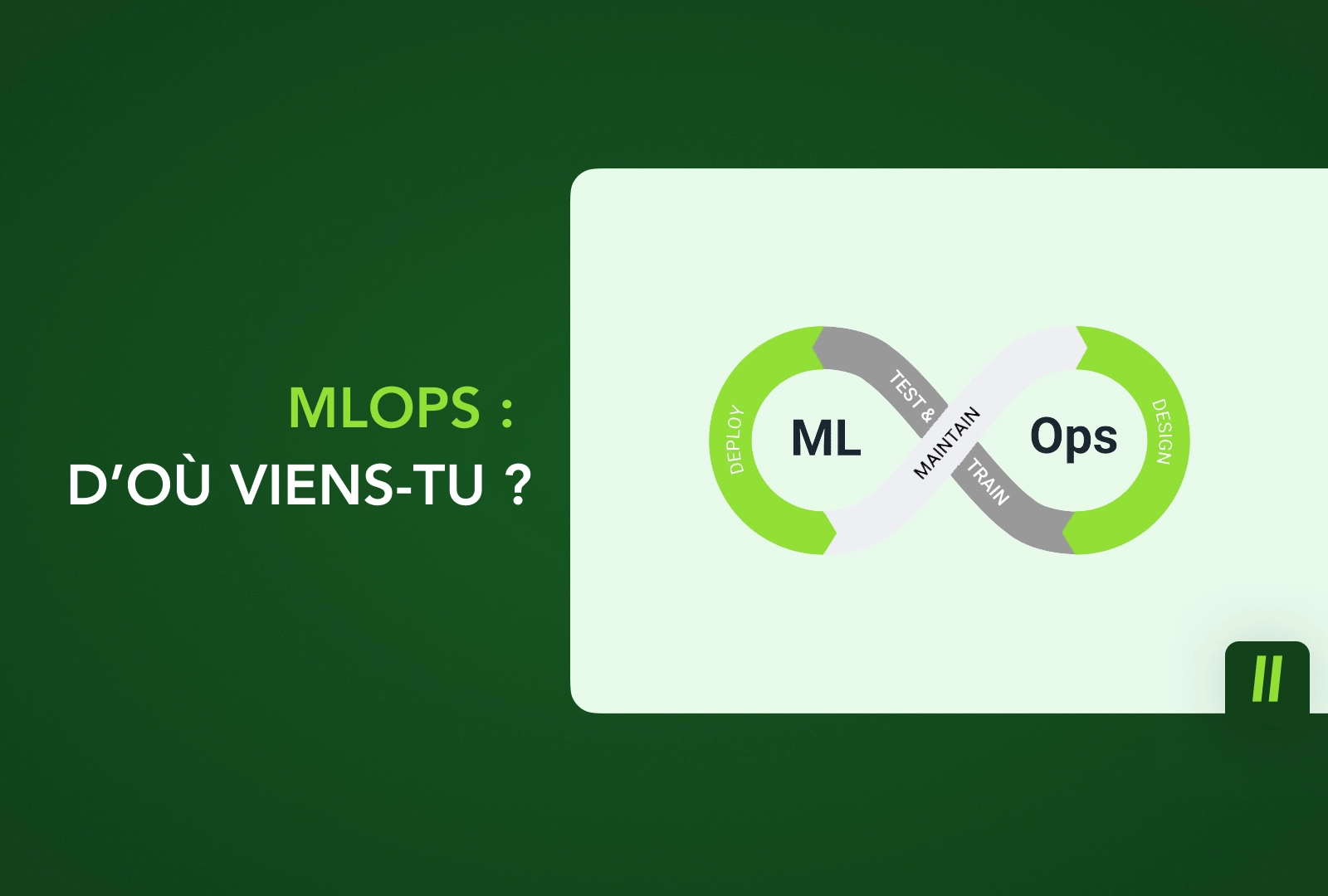
Bonnes pratiques de dév
Programmation
04/07/2025
Eric Dasse
04/07/2025
Eric Dasse
26/06/2025
Sylvain YVONNEAU
23/06/2025
Cyrille Martraire
14/05/2025
Alexis Nguyen-Goument
13/05/2025
Arnaud Lacroix
07/05/2025
Alexandra Courtiol
05/05/2025
Baptiste Macé
28/04/2025
12/08/2024
02/08/2024
02/08/2024
02/08/2024
Cyrille Martraire